Pub-Sub using MQ with Python
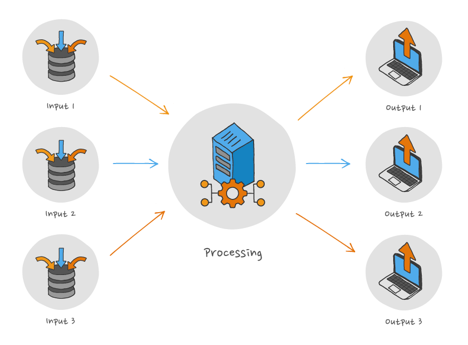
To use Python to publish and subscribe to a message queue (MQ), you can use a Python MQ client library such as pika
for RabbitMQ or google-cloud-pubsub
for Google Cloud Pub/Sub.
Here's an example using pika
to publish a message to a RabbitMQ queue:
import pika
# Establish a connection to the RabbitMQ server
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
# Declare the queue to publish the message to
queue_name = 'my_queue'
channel.queue_declare(queue=queue_name)
# Publish a message to the queue
message = 'Hello, World!'
channel.basic_publish(exchange='', routing_key=queue_name, body=message)
# Close the connection
connection.close()
In this example, we're using the pika
library to establish a connection to the RabbitMQ server and declare a queue called "my_queue". We then publish a message to the queue with the basic_publish
method, which takes the exchange, routing key, and message body as parameters.
To subscribe to messages from the queue, you can use the basic_consume
method to set up a consumer callback function that will receive messages as they arrive:
import pika
# Define the callback function to receive messages
def callback(ch, method, properties, body):
print(f'Received message: {body}')
# Establish a connection to the RabbitMQ server
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
# Declare the queue to consume messages from
queue_name = 'my_queue'
channel.queue_declare(queue=queue_name)
# Set up a consumer to receive messages from the queue
channel.basic_consume(queue=queue_name, on_message_callback=callback, auto_ack=True)
# Start consuming messages
channel.start_consuming()
In this example, we're defining a callback
function that will receive messages from the queue and print them to the console. We then establish a connection to the RabbitMQ server, declare the "my_queue" queue, and set up a consumer with basic_consume
that will call the callback
function to handle each incoming message.
Note that you'll need to adjust the connection parameters to match your RabbitMQ server configuration, and you may need to install the pika
library using pip
if you haven't already done so.
Similarly, you can use the google-cloud-pubsub
library to publish and subscribe to messages on Google Cloud Pub/Sub. The basic approach is similar to the pika
example above, but with different library-specific API calls and configuration settings.