How to do Control + A in selenium?
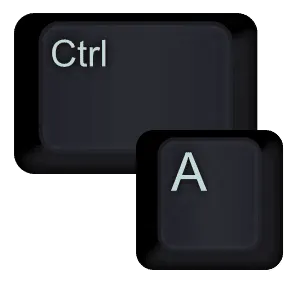
To send the "Control + A" key combination using Selenium in Java, you can use the sendKeys()
method on the element you want to send the keys to, and pass the Keys.chord()
method as the argument. The Keys.chord()
method allows you to combine multiple keys into a single action.
Here's an example:
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class Main {
public static void main(String[] args) {
// Set the path to the chromedriver executable
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
// Create a new ChromeDriver instance
WebDriver driver = new ChromeDriver();
// Navigate to the webpage with the input element
driver.get("https://example.com");
// Find the input element by ID
WebElement input = driver.findElement(By.id("myInput"));
// Send "Control + A" key combination to select all text in the input field
input.sendKeys(Keys.chord(Keys.CONTROL, "a"));
// Close the browser
driver.quit();
}
}
In this example, we first set the path to the chromedriver
executable using the System.setProperty()
method. We then create a new ChromeDriver
instance and navigate to the webpage with the input element using the get()
method.
Wethen use the findElement()
method to locate the input element on the page, using the By.id()
method to specify the ID of the element.
We then use the sendKeys()
method on the input element to send the "Control + A" key combination to select all text in the input field. The Keys.chord()
method is used to combine the Keys.CONTROL
and "a"
keys into a single action.
Note that this example assumes that the ID of the input element is myInput
. You should replace this with the appropriate ID for the input element you want to modify. Also, make sure to import the necessary classes (By
, WebDriver
, WebElement
, ChromeDriver
, Keys
) and to include the Selenium Java bindings in your project.