Dockerized with self-signed SSL
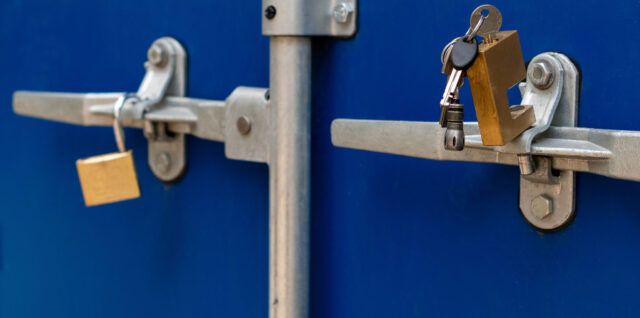
To add SSL support, we'll need to enable the Apache SSL module, generate an SSL certificate (self-signed for this example), and update the Apache configuration. Here's the updated Dockerfile:
# Stage 1: Composer
FROM composer:2 as composer
# Set work directory
WORKDIR /var/www/html
# Copy source code
COPY src /var/www/html
# Run composer init with default values
RUN composer init --name="" \
--description="" \
--type="" \
--license="" \
--author='n' \
--require="php:^7.4||^8.0" \
--stability="stable" \
--no-interaction
# Add AWS SDK and Flysystem S3 dependencies to composer.json
RUN composer require aws/aws-sdk-php:^3.263
# Stage 2: PHP
FROM php:8.2.5RC1-apache
# Enable Apache mod_rewrite and mod_ssl
RUN a2enmod rewrite && a2enmod ssl
# Install required system packages
RUN apt-get update && apt-get install -y \
git \
unzip \
libzip-dev \
openssl
# Install PHP extensions
RUN docker-php-ext-install zip
# Set work directory
WORKDIR /var/www/html
# Copy source code and installed dependencies from the composer stage
COPY --from=composer /var/www/html /var/www/html
# Generate a self-signed SSL certificate
RUN openssl req -x509 -nodes -newkey rsa:4096 -keyout /etc/ssl/private/ssl-cert-snakeoil.key -out /etc/ssl/certs/ssl-cert-snakeoil.pem -days 365 -subj "/C=US/ST=Unknown/L=Unknown/O=Unknown/CN=example.com" -sha256
# Update Apache configuration to enable SSL support
RUN echo '<VirtualHost *:80>' > /etc/apache2/sites-available/000-default.conf && \
echo ' ServerAdmin webmaster@localhost' >> /etc/apache2/sites-available/000-default.conf && \
echo ' DocumentRoot /var/www/html' >> /etc/apache2/sites-available/000-default.conf && \
echo ' ErrorLog ${APACHE_LOG_DIR}/error.log' >> /etc/apache2/sites-available/000-default.conf && \
echo ' CustomLog ${APACHE_LOG_DIR}/access.log combined' >> /etc/apache2/sites-available/000-default.conf && \
echo ' Redirect permanent / https://example.com/' >> /etc/apache2/sites-available/000-default.conf && \
echo '</VirtualHost>' >> /etc/apache2/sites-available/000-default.conf && \
echo '<IfModule mod_ssl.c>' > /etc/apache2/sites-available/default-ssl.conf && \
echo ' <VirtualHost _default_:443>' >> /etc/apache2/sites-available/default-ssl.conf && \
echo ' ServerAdmin webmaster@localhost' >> /etc/apache2/sites-available/default-ssl.conf && \
echo ' DocumentRoot /var/www/html' >> /etc/apache2/sites-available/default-ssl.conf && \
echo ' ErrorLog ${APACHE_LOG_DIR}/error.log' >> /etc/apache2/sites-available/default-ssl.conf && \
echo ' CustomLog ${APACHE_LOG_DIR}/ssl_access.log combined' >> /etc/apache2/sites-available/default-ssl.conf && \
echo ' SSLEngine on' >> /etc/apache2/sites-available/default-ssl.conf && \
echo ' SSLCertificateFile /etc/ssl/certs/ssl-cert-snakeoil.pem' >> /etc/apache2/sites-available/default-ssl.conf && \
echo ' SSLCertificateKeyFile /etc/ssl/private/ssl-cert-snakeoil.key' >> /etc/apache2/sites-available/default-ssl.conf && \
echo ' </VirtualHost>' >> /etc/apache2/sites-available/default-ssl.conf && \
echo '</IfModule>' >> /etc/apache2/sites-available/default-ssl.conf
# Enable the SSL site configuration
RUN a2ensite default-ssl
EXPOSE 80 443
This updated Dockerfile will enable SSL support, generate a self-signed SSL certificate, and configure Apache to use SSL. Note that self-signed certificates will cause a warning in browsers since they are not signed by a trusted certificate authority. In a production environment, you should obtain a certificate from a trusted certificate authority.